Stabilize raw ref macros
This stabilizes `raw_ref_macros` (https://github.com/rust-lang/rust/issues/73394), which is possible now that https://github.com/rust-lang/rust/issues/74355 is fixed.
However, as I already said in https://github.com/rust-lang/rust/issues/73394#issuecomment-751342185, I am not particularly happy with the current names of the macros. So I propose we also change them, which means I am proposing to stabilize the following in `core::ptr`:
```rust
pub macro const_addr_of($e:expr) {
&raw const $e
}
pub macro mut_addr_of($e:expr) {
&raw mut $e
}
```
The macro name change means we need another round of FCP. Cc `````@rust-lang/libs`````
Fixes#73394
Add `core::stream::Stream`
[[Tracking issue: #79024](https://github.com/rust-lang/rust/issues/79024)]
This patch adds the `core::stream` submodule and implements `core::stream::Stream` in accordance with [RFC2996](https://github.com/rust-lang/rfcs/pull/2996). The RFC hasn't been merged yet, but as requested by the libs team in https://github.com/rust-lang/rfcs/pull/2996#issuecomment-725696389 I'm filing this PR to get the ball rolling.
## Documentatation
The docs in this PR have been adapted from [`std::iter`](https://doc.rust-lang.org/std/iter/index.html), [`async_std::stream`](https://docs.rs/async-std/1.7.0/async_std/stream/index.html), and [`futures::stream::Stream`](https://docs.rs/futures/0.3.8/futures/stream/trait.Stream.html). Once this PR lands my plan is to follow this up with PRs to add helper methods such as `stream::repeat` which can be used to document more of the concepts that are currently missing. That will allow us to cover concepts such as "infinite streams" and "laziness" in more depth.
## Feature gate
The feature gate for `Stream` is `stream_trait`. This matches the `#[lang = "future_trait"]` attribute name. The intention is that only the APIs defined in RFC2996 will use this feature gate, with future additions such as `stream::repeat` using their own feature gates. This is so we can ensure a smooth path towards stabilizing the `Stream` trait without needing to stabilize all the APIs in `core::stream` at once. But also don't start expanding the API until _after_ stabilization, as was the case with `std::future`.
__edit:__ the feature gate has been changed to `async_stream` to match the feature gate proposed in the RFC.
## Conclusion
This PR introduces `core::stream::{Stream, Next}` and re-exports it from `std` as `std::stream::{Stream, Next}`. Landing `Stream` in the stdlib has been a mult-year process; and it's incredibly exciting for this to finally happen!
---
r? `````@KodrAus`````
cc/ `````@rust-lang/wg-async-foundations````` `````@rust-lang/libs`````
BTreeMap: prevent tree from ever being owned by non-root node
This introduces a new marker type, `Dying`, which is used to note trees which are in the process of deallocation. On such trees, some fields may be in an inconsistent state as we are deallocating the tree. Unfortunately, there's not a great way to express conditional unsafety, so the methods for traversal can cause UB if not invoked correctly, but not marked as such. This is not a regression from the previous state, but rather isolates the destructive methods to solely being called on the dying state.
Trying to shrink_to greater than capacity should be no-op
Per the discussion in https://github.com/rust-lang/rust/issues/56431, `shrink_to` shouldn't panic if you try to make a vector shrink to a capacity greater than its current capacity.
BTreeMap: test all borrowing interfaces and test more chaotic order behavior
Inspired by #81169, test what happens if you mess up order of the type with which you search (as opposed to the key type).
r? `@Mark-Simulacrum`
BTreeMap: bring back the key slice for immutable lookup
Pave the way for binary search, by reverting a bit of #73971, which banned `keys` for misbehaving while it was defined for every `BorrowType`. Adding some `debug_assert`s along the way.
r? `@Mark-Simulacrum`
mark raw_vec::ptr with inline
when a lot of vectors is used in a enum as in the example in #66617 if this function is not inlined and multiple cgus is used this results in huge compile times. with this fix the compile time is 6s from minutes for the example in #66617. I did not have the patience to wait for it to compile for more then 3 min.
Add doc aliases for memory allocations
This patch adds doc aliases for various C allocation functions, making it possible to search for the C-equivalent of a function and finding the (safe) Rust counterpart:
- `Vec::with_capacity` / `Box::new` / `vec!` -> alloc + malloc, allocates memory
- `Box::new_zeroed` -> calloc, allocates zeroed-out memory
- `Vec::{reserve,reserve_exact,try_reserve_exact,shrink_to_fit,shrink_to}` -> realloc, reallocates a previously allocated slice of memory
It's worth noting that `Vec::new` does not allocate, so we don't link to it. Instead people are probably looking for `Vec::with_capacity` or `vec!`. I hope this will allow people comfortable with the system allocation APIs to make it easier to find what they may be looking for.
Thanks!
Enforce statically that `MIN_NON_ZERO_CAP` is calculated at compile time
Previously, it would usually get computed by LLVM, but this enforces it. This removes the need for the comment saying "LLVM is smart enough".
I don't expect this to make a performance difference, but I do think it makes the performance properties easier to reason about.
Fix broken links with `--document-private-items` in the standard library
As it was suggested in #81037 `SpecFromIter` is not
in the scope and therefore we get a warning when we try to
do document private intems in `rust/library/alloc/`.
This addresses #81037 by adding the trait in the scope as ```@jyn514```
suggested and also adding an `allow(unused_imports)` flag so that
the compiler does not complain, Since the trait is not used
per se in the code, it's just needed to have properly documented
docs.
Improve grammar in documentation of format strings
The docs previously were
* using some weird `<` and `>` around some nonterminals
* _correct me if these **did** have any meaning_
* using of a (not explicitly defined) `text` nonterminal that didn’t explicitly disallow productions containing `'{'` or `'}'`
* incorrect in not allowing for `x?` and `X?` productions of `type`
* unnecessarily ambiguous, both
* allowing `type` to be `''`, and
* using an optional `[type]`
* using inconsistent underscore/hyphenation style between `format_string` and `format_spec` vs `maybe-format`
_Rendered:_
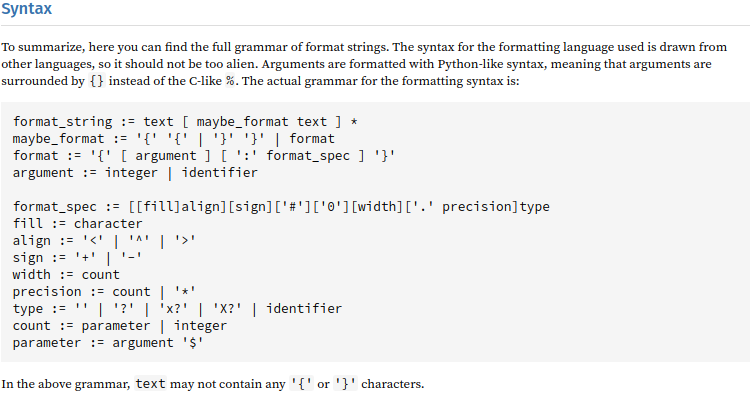
_(current docs: https://doc.rust-lang.org/nightly/std/fmt/#syntax)_
```@rustbot``` modify labels: T-doc
Visualize vector while differentiating between stack and heap.
Inspired by cheats.rs, as this is probably the first place beginner go,
they could understand stack and heap, length and capacity with this. Not
sure if adding this means we should add to other places too.
Superseeds #76066
BTreeMap: prefer bulk_steal functions over specialized ones
The `steal_` functions (apart from their return value) are basically specializations of the more general `bulk_steal_` functions. This PR removes the specializations. The library/alloc benchmarks say this is never slower and up to 6% faster.
r? ``@Mark-Simulacrum``
As it was suggested in #81037 `SpecFromIter` is not
in the scope and therefore (even it should fail),
we get a warning when we try do document private
intems in `rust/library/alloc/`.
This fixes#81037 by adding the trait in the scope
and also adding an `allow(unused_imports)` flag so that
the compiler does not complain, Since the trait is not used
per se in the code, it's just needed to have properly documented
docs.
Don't make tools responsible for checking unknown and renamed lints
Previously, clippy (and any other tool emitting lints) had to have their
own separate UNKNOWN_LINTS pass, because the compiler assumed any tool
lint could be valid. Now, as long as any lint starting with the tool
prefix exists, the compiler will warn when an unknown lint is present.
This may interact with the unstable `tool_lint` feature, which I don't entirely understand, but it will take the burden off those external tools to add their own lint pass, which seems like a step in the right direction to me.
- Don't mark `ineffective_unstable_trait_impl` as an internal lint
- Use clippy's more advanced lint suggestions
- Deprecate the `UNKNOWN_CLIPPY_LINTS` pass (and make it a no-op)
- Say 'unknown lint `clippy::x`' instead of 'unknown lint x'
This is tested by existing clippy tests. When https://github.com/rust-lang/rust/pull/80527 merges, it will also be tested in rustdoc tests. AFAIK there is no way to test this with rustc directly.
Force vec![] to expression position only
r? `@oli-obk`
I went with the lazy way of only changing what broke. I moved the test to ui/macros because the diagnostics no longer give suggestions.
Closes#61933
BTreeMap: expose new_internal function and sanitize from_new_internal
`new_internal` is the functional core of the imperative `push_internal_level`, and `from_new_internal` can easily do a proper job instead of returning a half-baked node.
r? `@Mark-Simulacrum`
Re-stabilize Weak::as_ptr and friends for unsized T
As per [T-lang consensus](https://hackmd.io/7r3_is6uTz-163fsOV8Vfg), this uses a branch to handle the dangling case. The discussed optimization of only doing the branch in the T: ?Sized case is left for a followup patch, as doing so is not trivial (as it requires specialization) and not _obviously_ better (as it requires using `wrapping_offset` rather than `offset` more).
<details><summary>Basically said optimization</summary>
Specialize on `T: Sized`:
```rust
fn as_ptr(&self) -> *const T {
if [ T is Sized ] || !is_dangling(ptr) {
(ptr as *mut T).set_ptr_value( (ptr as *mut u8).wrapping_offset(data_offset) )
} else {
ptr::null()
}
}
fn from_raw(*const T) -> Self {
if [ T is Sized ] || !ptr.is_null() {
let ptr = (ptr as *mut RcBox).set_ptr_value( (ptr as *mut u8).wrapping_offset(-data_offset) );
Weak { ptr }
} else {
Weak::new()
}
}
```
(but with more `set_ptr_value` to avoid `Sized` restrictions and maintain metadata.)
Written in this fashion, this is not a correctness-critical specialization (i.e. so long as `[ T is Sized ]` is false for unsized `T`, it can be `rand()` for sized `T` without breaking correctness), but it's still touchy, so I'd rather do it in another PR with separate review.
---
</details>
This effectively reverts #80422 and re-establishes #74160. T-libs [previously signed off](https://github.com/rust-lang/rust/pull/74160#issuecomment-660539373) on this stable API change in #74160.
Clarify what the effects of a 'logic error' are
This clarifies what a 'logic error' is (which is a term used to describe what happens if you put things in a hash table or btree and then use something like a refcell to break the internal ordering). This tries to be as vague as possible, as we don't really want to promise what happens, except "bad things, but not UB". This was discussed in #80657