Add a chapter on the test harness.
There isn't really any online documentation on the test harness, so this adds a chapter to the rustc book which provides information on how the harness works and details on the command-line options.
Revert LLVM D81803 because it broke Windows 7
This submodule update reverts <https://reviews.llvm.org/D81803>.
While that change is meant to fix a real bug, [LLVM PR42623], it caused
new permission errors on Windows 7 that make it unable to build any
archives. This is probably the same root cause as [LLVM PR48378].
Fixes#81051. We'll file a new Rust issue to track the LLVM resolution.
[LLVM PR42623]: https://bugs.llvm.org/show_bug.cgi?id=42623
[LLVM PR48378]: https://bugs.llvm.org/show_bug.cgi?id=48378
Remove the x86_64-rumprun-netbsd target
Herein we remove the target from the compiler and the code from libstd intended to support the now-defunct rumprun project.
Closes#81514
Rollup of 11 pull requests
Successful merges:
- #81856 (Suggest character encoding is incorrect when encountering random null bytes)
- #82395 (Add missing "see its documentation for more" stdio)
- #82401 (Remove a redundant macro)
- #82498 (Use log level to control partitioning debug output)
- #82534 (Link crtbegin/crtend on musl to terminate .eh_frame)
- #82537 (Update measureme dependency to the latest version)
- #82561 (doc: cube root, not cubic root)
- #82563 (Fix intra-doc handling of `Self` in enum)
- #82584 (Add ARIA role to sidebar toggle in Rustdoc)
- #82596 (clarify RW lock's priority gotcha)
- #82607 (Add a getter for Frame.loc)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
clarify RW lock's priority gotcha
In particular, the following program works on Linux, but deadlocks on
mac:
```rust
use std::{
sync::{Arc, RwLock},
thread,
time::Duration,
};
fn main() {
let lock = Arc::new(RwLock::new(()));
let r1 = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _rg = lock.read();
eprintln!("r1/1");
sleep(1000);
let _rg = lock.read();
eprintln!("r1/2");
sleep(5000);
}
});
sleep(100);
let w = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _wg = lock.write();
eprintln!("w");
}
});
sleep(100);
let r2 = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _rg = lock.read();
eprintln!("r2");
sleep(2000);
}
});
r1.join().unwrap();
r2.join().unwrap();
w.join().unwrap();
}
fn sleep(ms: u64) {
std:🧵:sleep(Duration::from_millis(ms))
}
```
Context: I was completely mystified by a my CI deadlocking on mac ([here](https://github.com/matklad/xshell/pull/7)), until ``@azdavis`` debugged the issue. See a stand-alone reproduciton here: https://github.com/matklad/xshell/pull/15
Add ARIA role to sidebar toggle in Rustdoc
This indicates that the div is an interactive element, and makes the sidebar toggle "clickable" in assistive technologies.
Example of Vimium after this change has been applied (see the issue mentioned below for a screenshot of before):
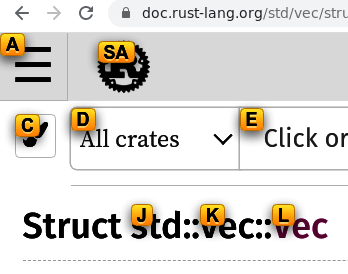
Fixes#82582
Update measureme dependency to the latest version
This version adds the ability to use `rdpmc` hardware-based performance
counters instead of wall-clock time for measuring duration. This also
introduces a dependency on the `perf-event-open-sys` crate on Linux
which is used when using hardware counters.
r? ```@oli-obk```
Link crtbegin/crtend on musl to terminate .eh_frame
For some targets, rustc uses a "CRT fallback", where it links CRT
object files it ships instead of letting the host compiler link
them.
On musl, rustc currently links crt1, crti and crtn (provided by
libc), but does not link crtbegin and crtend (provided by libgcc).
In particular, crtend is responsible for terminating the .eh_frame
section. Lack of terminator may result in segfaults during
unwinding, as reported in #47551 and encountered by the LLVM 12
update in #81451.
This patch links crtbegin and crtend for musl as well, following
the table at the top of crt_objects.rs.
r? ``@nagisa``
Add missing "see its documentation for more" stdio
StdoutLock and StderrLock does not have example, it would be better
to leave "see its documentation for more" like iter docs.
Suggest character encoding is incorrect when encountering random null bytes
This adds a note whenever null bytes are seen at the start of a token unexpectedly, since those tend to come from UTF-16 encoded files without a [BOM](https://en.wikipedia.org/wiki/Byte_order_mark) (if a UTF-16 BOM appears it won't be valid UTF-8, but if there is no BOM it be both valid UTF-16 and valid but garbled UTF-8). This approach was suggested in https://github.com/rust-lang/rust/issues/73979#issuecomment-653976451.
Closes#73979.
Skip Ty w/o infer ty/const in trait select
Remove some allocations & also add `skip_current_subtree` to skip subtrees with no inferred items.
r? `@eddyb` since marked in the FIXME
In particular, the following program works on Linux, but deadlocks on
mac:
use std::{
sync::{Arc, RwLock},
thread,
time::Duration,
};
fn main() {
let lock = Arc::new(RwLock::new(()));
let r1 = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _rg = lock.read();
eprintln!("r1/1");
sleep(1000);
let _rg = lock.read();
eprintln!("r1/2");
sleep(5000);
}
});
sleep(100);
let w = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _wg = lock.write();
eprintln!("w");
}
});
sleep(100);
let r2 = thread::spawn({
let lock = Arc::clone(&lock);
move || {
let _rg = lock.read();
eprintln!("r2");
sleep(2000);
}
});
r1.join().unwrap();
r2.join().unwrap();
w.join().unwrap();
}
fn sleep(ms: u64) {
std:🧵:sleep(Duration::from_millis(ms))
}
Specialize slice::fill with Copy type and u8/i8/bool
I don't expect rustperf could measure any perf improvements with this changes
since `slice::fill` is newly added.
Godbolt link for this change: <https://rust.godbolt.org/z/r3fzee>.
r? `@matthewjasper` since this patch added new specialization.
Combine HasAttrs and HasTokens into AstLike
When token-based attribute handling is implemeneted in #80689,
we will need to access tokens from `HasAttrs` (to perform
cfg-stripping), and we will to access attributes from `HasTokens` (to
construct a `PreexpTokenStream`).
This PR merges the `HasAttrs` and `HasTokens` traits into a new
`AstLike` trait. The previous `HasAttrs` impls from `Vec<Attribute>` and `AttrVec`
are removed - they aren't attribute targets, so the impls never really
made sense.
When token-based attribute handling is implemeneted in #80689,
we will need to access tokens from `HasAttrs` (to perform
cfg-stripping), and we will to access attributes from `HasTokens` (to
construct a `PreexpTokenStream`).
This PR merges the `HasAttrs` and `HasTokens` traits into a new
`AstLike` trait. The previous `HasAttrs` impls from `Vec<Attribute>` and `AttrVec`
are removed - they aren't attribute targets, so the impls never really
made sense.
Rollup of 14 pull requests
Successful merges:
- #81794 (update tracking issue for `relaxed_struct_unsize`)
- #82057 (Replace const_cstr with cstr crate)
- #82370 (Improve anonymous lifetime note to indicate the target span)
- #82394 (⬆️ rust-analyzer)
- #82396 (Add Future trait for doc_spotlight feature doc)
- #82404 (Test hexagon-enum only when llvm target is present)
- #82419 (expand: Preserve order of inert attributes during expansion)
- #82420 (Enable API documentation for `std::os::wasi`.)
- #82421 (Add a `size()` function to WASI's `MetadataExt`.)
- #82442 (Skip emitting closure diagnostic when closure_kind_origins has no entry)
- #82473 (Use libc::accept4 on Android instead of raw syscall.)
- #82482 (Use small hash set in `mir_inliner_callees`)
- #82490 (Update cargo)
- #82494 (Substitute erased lifetimes on bad placeholder type)
Failed merges:
- #82448 (Combine HasAttrs and HasTokens into AstLike)
r? `@ghost`
`@rustbot` modify labels: rollup
Update cargo
11 commits in bf5a5d5e5d3ae842a63bfce6d070dfd438cf6070..572e201536dc2e4920346e28037b63c0f4d88b3c
2021-02-18 15:49:14 +0000 to 2021-02-24 16:51:20 +0000
- Pass the error message format to rustdoc (rust-lang/cargo#9128)
- Fix test target_in_environment_contains_lower_case (rust-lang/cargo#9203)
- Fix hang on broken stderr. (rust-lang/cargo#9201)
- Make it more clear which module is being tested when running cargo test (rust-lang/cargo#9195)
- Updates to edition handling. (rust-lang/cargo#9184)
- Add --cfg and --rustc-cfg flags to output compiler configuration (rust-lang/cargo#9002)
- Run rustdoc doctests relative to the workspace (rust-lang/cargo#9105)
- Add support for [env] section in .cargo/config.toml (rust-lang/cargo#9175)
- Add schema field and `features2` to the index. (rust-lang/cargo#9161)
- Document the default location where cargo install emitting build artifacts (rust-lang/cargo#9189)
- Do not exit prematurely if anything failed installing. (rust-lang/cargo#9185)
Use small hash set in `mir_inliner_callees`
Use small hash set in `mir_inliner_callees` to avoid temporary
allocation when possible and quadratic behaviour for large number of
callees.
Use libc::accept4 on Android instead of raw syscall.
This PR replaces the use of a raw `accept4` syscall with `libc::accept4`. This was originally added (by me) because `std` couldn't update to the latest `libc` with `accept4` support for android. By now, libc is already on 0.2.85, so the workaround can be removed.
`@rustbot` label +O-android +T-libs-impl
Add a `size()` function to WASI's `MetadataExt`.
WASI's `filestat` type includes a size field, so expose it in
`MetadataExt` via a `size()` function, similar to the corresponding Unix
function.
r? ``````@alexcrichton``````
Enable API documentation for `std::os::wasi`.
This adds API documentation support for `std::os::wasi` modeled after
how `std::os::unix` works, so that WASI can be documented [here] along
with the other platforms.
[here]: https://doc.rust-lang.org/stable/std/os/index.html
Two changes of particular interest:
- This changes the `AsRawFd` for `io::Stdin` for WASI to return
`libc::STDIN_FILENO` instead of `sys::stdio::Stdin.as_raw_fd()` (and
similar for `Stdout` and `Stderr`), which matches how the `unix`
version works. `STDIN_FILENO` etc. may not always be explicitly
reserved at the WASI level, but as long as we have Rust's `std` and
`libc`, I think it's reasonable to guarantee that we'll always use
`libc::STDIN_FILENO` for stdin.
- This duplicates the `osstr2str` utility function, rather than
trying to share it across all the configurations that need it.
r? ```@alexcrichton```
Improve anonymous lifetime note to indicate the target span
Improvement for #81650
Cc #81995
Message after this improvement:
(Improve note in the middle)
```
error[E0311]: the parameter type `T` may not live long enough
--> src/main.rs:25:11
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| -- help: consider adding an explicit lifetime bound...: `T: 'a +`
25 | scope.spawn(move |_| {
| ^^^^^
|
note: the parameter type `T` must be valid for the anonymous lifetime defined on the function body at 24:40...
--> src/main.rs:24:40
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| ^^^^^
note: ...so that the type `[closure@src/main.rs:25:17: 27:6]` will meet its required lifetime bounds
--> src/main.rs:25:11
|
25 | scope.spawn(move |_| {
| ^^^^^
```
r? ``````@estebank``````
Replace const_cstr with cstr crate
This PR replaces the `const_cstr` macro inside `rustc_data_structures` with `cstr` macro from [cstr](https://crates.io/crates/cstr) crate.
The two macros basically serve the same purpose, which is to generate `&'static CStr` from a string literal. `cstr` is better because it validates the literal at compile time, while the existing `const_cstr` does it at runtime when `debug_assertions` is enabled. In addition, the value `cstr` generates can be used in constant context (which is seemingly not needed anywhere currently, though).